Log4Net is a popular logging framework, and if you have an existing application that you wish to move to Azure compute, you probably want to avoid rewriting your application to use another logging framework. Luckily, keeping Log4Net as your logging tool in Azure is certainly possible, but there are a few hoops you have to jump through to get there.
There are several ways to achieve this goal. I decided to rely as much as possible on a feature provided in Azure Compute that allows for automatically synchronizing certain directories on the instance’s local file system to Azure blob storage. Using this approach, there are only very few changes that need to be done in the application, and indeed none of the existing code needs to be altered.
Baseline: an existing application which uses Log4Net
In order for this example to work, we need an application that we what to move to the cloud:
- Start off with
File->New->Project...
in Visual Studio and use the “ASP.NET Web Application” template - Add Log4Net capabilities to the application. This can be done by adding a reference to Log4Net using Nuget, and then configuring it like Phil Haack has described here.
Then, run the web application locally in Visual Studio to assert that the logging works.
Enabling the application for Azure
Starting off with the simple ASP.NET web application we created in the previous section, do the following:
- Right-click on the solution in Visual Studio to select
Add->New Project
. Use the Windows Azure Project template, and do not add any roles in the dialog box initially. - Set the newly created cloud project as the startup project in the solution.
- Right-click on the Roles-folder of the newly created Azure project and select
Add->Web role project in solution...
to add the web application project as an Azure Web role.
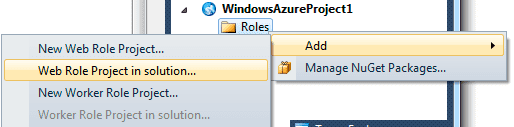
Now, press F5
to run the application in the local Azure development environment (DevFabric) to see that it works (functionally, not logging-wise)
So, we are done with the prerequisites. Now to the interesting parts!
Setting log directory for Azure
The first issue we will grapple with is the fact that in Azure Compute, the application effectively runs in a sandbox with limited access to the file system, which means that the “standard” approach logging to a file does not work. Basically, the Azure compute role has only access to a certain subdirectory of the file system and the exact location needs to be retrieved by the application at runtime.
In order to retain the existing logging in the application, locating the path to the role’s designated area on the disk can be solved by subclassing one of the appenders that Log4Net provides out of the box. I chose the RollingFileAppender
because it provides the ability to split the log into several files. This is beneficial from an operations perspective. Here’s what the custom appender looks like:
using System.Diagnostics;
using System.IO;
using log4net.Appender;
using Microsoft.WindowsAzure.ServiceRuntime;
namespace Demo.Log4Net.Azure
{
public class AzureAppender : RollingFileAppender
{
public override string File
{
set
{
base.File = RoleEnvironment.GetLocalResource("Log4Net").RootPath + @""
+ new FileInfo(value).Name + "_"
+ Process.GetCurrentProcess().ProcessName;
}
}
}
}
What happens here, is that when the configuration is read when the logging framework initializes, it calls our method to set the log file name. This corresponds to the file element in the XML configuration for the appender:
<log4net>
<appender>
<param name="File" value="app.log" />
...
</appender>
...
</log4net>
What happens here, is that the application asks the role environment for the whereabouts of the local resource called “Log4Net”. This resource is a directory that we specify designated for containing our logs and needs to be specified in the ServiceDefinition.csdef
file:
<ServiceDefinition>
<WebRole name="WebRole1">
<LocalResources>
<LocalStorage name="Log4Net" sizeInMB="2048" cleanOnRoleRecycle="true"/>
</LocalResources>
</WebRole>
</ServiceDefinition>
When we have the path of the local resource, it is used to construct an absolute path for the log file. Also note that the current process name is appended to the filename. This is done because if you run the application as a WebRole in “Full IIS” mode in Azure, the web application and the RoleEntryPoint
code run in different processes. (If you look at blog entries on the Internet for Azure information, you should have in mind that the “Full IIS” mode was introduced with the Azure SDK version 1.3 in late 2010, and information that predates this might not be valid for the current Azure version.) This means that if there are log entries in the RoleEntryPoint
as well as in the rest of your application, two processes would potentially try to keep a write lock on the file at the same time. Therefore, we use one log file for each process. Note that this is not a relevant topic for Worker roles. For more on the execution model, take a look here.
So, now that the new custom appender is ready, we need to change the Log4Net configuration to use it. Basically, we change the assembly type in the appender configuration section so that the configuration looks like this:
<log4net>
<appender name="AzureRollingLogFileAppender" type="Demo.Log4Net.Azure.AzureAppender, Demo.Log4Net.Azure">
<param name="File" value="app.log" />
<param name="AppendToFile" value="true" />
<param name="RollingStyle" value="Date" />
<param name="StaticLogFileName" value="false" />
<param name="DatePattern" value=".yyyy-MM-dd.log" />
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date [%thread] %-5level %logger [%appdomain] - %message%newline" />
</layout>
</appender>
<root>
<level value="DEBUG" />
<appender-ref ref="AzureRollingLogFileAppender" />
</root>
</log4net>
Now it’s time to run the application to see if the logging works. First, deploy to devfabric, and then open the Windows Azure Compute Emulator. Right-click on the running instance, and click on Open local store...
.
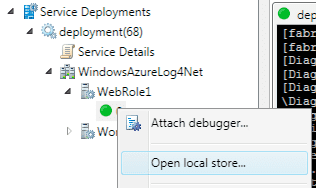
Then navigate to the ‘directoryLog4Net
‘ to find the log files:
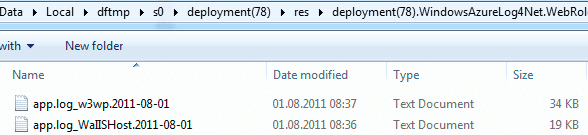
Persisting logs to Azure blob storage
The next issue we need to handle, is the fact that the local file system in an Azure role instance is not persistent. Local data will be lost when the application is redeployed (and also when the Role recycles, if you have chosen to do so). Furthermore, the only way to access the local file system is using a Remote Desktop Connection. In theory, you could probably also make the directory a shared drive accessible over the Internet, but you probably would not want to do that. Besides, it will be a headache if you have a lot of instances.
So, the solution that Azure offers to this, is to have a scheduled synchronization of certain of the local resources (directories) to the Azure blob store. What we need to do, is to add the following code to the descendant of RoleEntryPoint
:
public class WebRole : RoleEntryPoint
{
public override bool OnStart()
{
var diagnosticsConfig = DiagnosticMonitor.GetDefaultInitialConfiguration();
diagnosticsConfig.Directories.ScheduledTransferPeriod = TimeSpan.FromMinutes(5);
diagnosticsConfig.Directories.DataSources.Add(
new DirectoryConfiguration
{
Path = RoleEnvironment.GetLocalResource("Log4Net").RootPath,
DirectoryQuotaInMB = 2048,
Container = "wad-log4net"
}
);
DiagnosticMonitor.Start("Microsoft.WindowsAzure.Plugins.Diagnostics.ConnectionString", diagnosticsConfig);
return base.OnStart();
}
}
…and that’s it. Now you can try to run the application and observe a container called ‘wad-log4net’ will be created in your blob service account that will contain the logs:
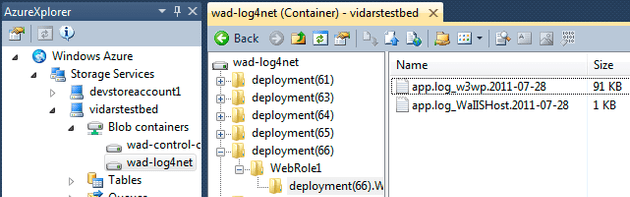
(I use the AzureXplorer extension for Visual Studio.)
The solution shown here targeted an ASP.NET application running as a WebRole, but the setup works equally well for Worker roles.